Stimulation parameter loader#
Overview#
The StimParamLoader
class is a small utility for managing stimulation parameters and previewing them in a plot. It provides functionalities to validate, enable, disable, and send stimulation parameters to the Intan software.
To install this utiiity, run the following command :
pip install git+https://github.com/FinalSpark-np/np-utils.git#egg=np_utils[SPL]
Caution
Neuroplaform access is required to use this utility. This utility requires python 3.11. If you need help upgrading python on your provided Neuroplatform machine, please contact us.
Usage#
Importing the utility#
from np_utils.parameters_loader import StimParamLoader
Creating a StimParamLoader#
To create a StimParamLoader
instance, use the following :
stimparams
: List ofStimParam
instances. Can be None or empty if you prefer to modify the parameters later.intan
: Intan software instance. Can be None if you have not booked the Intan, or do not want to send parameters immediately.must_connect
: If True, raise an error if the Intan is not connected. Defaults to False.verbose
: If True, print out log messages. Defaults to True. Recommended to use while you are first using the utility to understand the process.
Changing parameters#
There are several options to change the parameters :
Create the instance with a list of
StimParam
instances.Modify the
stimparams
attribute directly.Use the
add_stimparam
method to add a newStimParam
instance to the list.Use the
reset
method to clear all parameters.
Danger
Remember to always disable ALL parameters sent. If you remove or modify the list of parameters, make sure to disable all parameters and send the update to the Intan before doing so.
If you do not disable all parameters before sending new ones, there may be old leftover parameters that are still enabled, which can cause unexpected stimulation when sending triggers.
If you suspect there may be leftover parameters either from your code or another user’s session, please contact us.
Previewing parameters#
To preview the parameters in a plot, use the preview_parameters
method. You can choose to hide empty parameters and use a colorblind-friendly color scheme.
The plot is interactive and will display the settings for each parameter when you hover over the electrode.
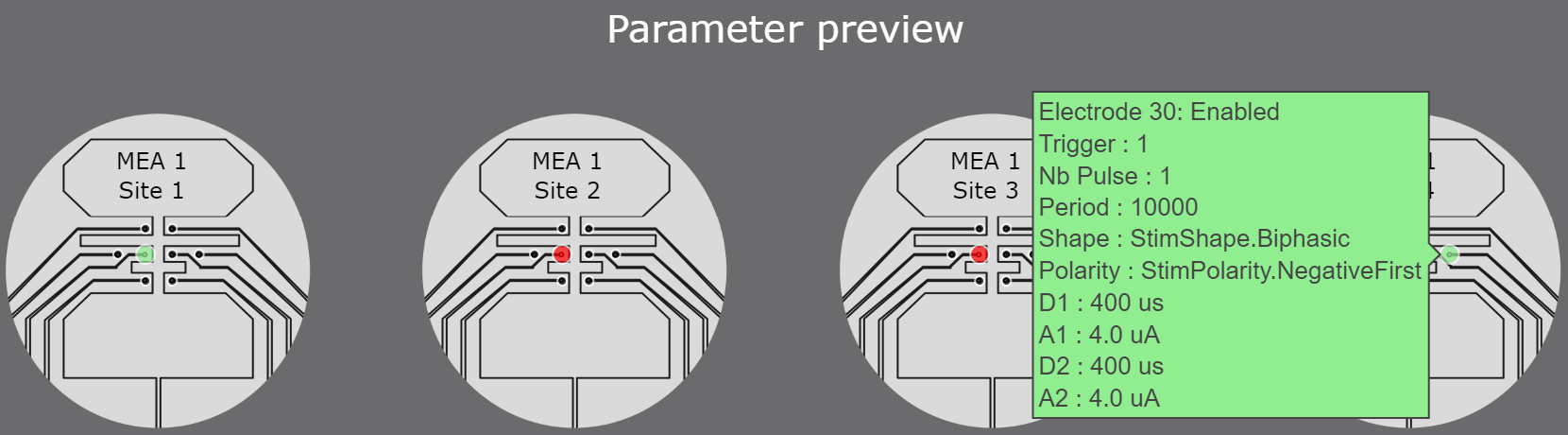
Fig. 13 StimParamLoader plot preview#
Sending parameters#
To send the parameters to the Intan, use the send_parameters
method.
This will check if all parameters are valid and enabled before sending them to the Intan.
When you are finished with this set of parameters, remember to disable all parameters and send the update to the Intan, using the disable_all_and_send
method.
Logging messages#
If using the verbose
option, all log messages will be shown.
If this is impractical to show during your experiment, for example if you are changing parameters frequently in a loop, you may set verbose=False
.
Hint
To get a history of all log messages, use the get_log
method to return a DataFrame of all messages, even if verbose=False
.
Example code#
from np_utils.parameters_loader import StimParamLoader
from neuroplatform import StimParam, StimPolarity
stimparam1 = StimParam()
stimparam2 = StimParam()
stimparam3 = StimParam()
stimparam4 = StimParam()
###
stimparam1.index = 2
stimparam1.enable = True
stimparam1.trigger_key = 0
stimparam1.nb_pulse = 1
stimparam1.polarity = StimPolarity.PositiveFirst
stimparam1.phase_duration1 = 100
stimparam1.phase_duration2 = 100
stimparam1.phase_amplitude1 = 1.0
stimparam1.phase_amplitude2 = 1.0
###
stimparam2.index = 10
stimparam2.enable = False
stimparam2.trigger_key = 0
stimparam2.nb_pulse = 1
stimparam2.polarity = StimPolarity.NegativeFirst
stimparam2.phase_duration1 = 200
stimparam2.phase_duration2 = 200
stimparam2.phase_amplitude1 = 2.0
stimparam2.phase_amplitude2 = 2.0
###
stimparam3.index = 18
stimparam3.enable = False
stimparam3.trigger_key = 0
stimparam3.nb_pulse = 1
stimparam3.polarity = StimPolarity.PositiveFirst
stimparam3.phase_duration1 = 300
stimparam3.phase_duration2 = 300
stimparam3.phase_amplitude1 = 3.0
stimparam3.phase_amplitude2 = 3.0
###
stimparam4.index = 30
stimparam4.enable = True
stimparam4.trigger_key = 1
stimparam4.nb_pulse = 1
stimparam4.polarity = StimPolarity.NegativeFirst
stimparam4.phase_duration1 = 400
stimparam4.phase_duration2 = 400
stimparam4.phase_amplitude1 = 4.0
stimparam4.phase_amplitude2 = 4.0
# Create a list of stimparams
stimparams = [stimparam1, stimparam2, stimparam3, stimparam4]
loader = StimParamLoader(
stimparams, intan=None, verbose=False
) # not connecting to Intan.
# If you want to try the Intan, make sure to book, and set intan=IntanSofware()
# Preview the stimparams with an interactive plot
loader.preview_parameters(colorblind=False)
# Enabling all stimparams (this can be done safely without booking the Intan)
loader.enable_all()
# Make sure to book the system before enabling the stimparams
# loader.send_parameters()
# Disable all stimparams
# loader.disable_all_and_send()
# Show the log
loader.get_log()